阅读(3893)
赞(0)
Javascript对象继承
2017-01-06 19:14:37 更新
Javascript面向对象设计 - Javascript对象继承
要在对象之间添加继承,指定什么对象应该是新对象“s [[Prototype]]。
要在对象之间添加继承,指定什么对象应该是新对象“s [[Prototype]]。...
我们可以使用Object.create()方法显式指定[[Prototype]]。
我们可以使用Object.create()方法显式指定[[Prototype]]。...
第一个参数是在新对象中用于[[Prototype]]的对象。
第一个参数是在新对象中用于[[Prototype]]的对象。...
例子
以下代码显示了如何在对象之间创建继承。
var book1 = {
name : "Javascript",
writeLine : function() { //from w w w . j a va2s . c om
console.log(this.name);
}
};
var book2 = Object.create(book1, {
name : {
configurable : true,
enumerable : true,
value : "CSS",
writable : true
}
});
book1.writeLine(); // outputs "Javascript"
book2.writeLine(); // outputs "CSS"
console.log(book1.hasOwnProperty("writeLine")); // true
console.log(book1.isPrototypeOf(book2)); // true
console.log(book2.hasOwnProperty("writeLine")); // false
上面的代码生成以下结果。
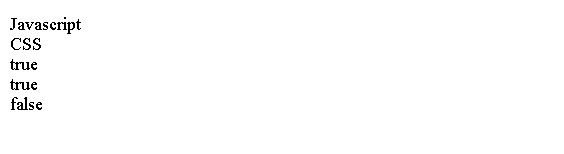
注意
要访问超类型方法,我们可以直接访问超类型的方法原型并使用call()或apply()在子类型对象上执行方法。
function Rectangle(length, width) {
this.length = length; /*from w w w .ja v a2 s . com*/
this.width = width;
}
Rectangle.prototype.getArea = function() {
return this.length * this.width;
};
Rectangle.prototype.toString = function() {
return "[Rectangle " + this.length + "x" + this.height + "]";
};
// inherits from Rectangle
function Square(size) {
Rectangle.call(this, size, size);
}
Square.prototype = Object.create(Rectangle.prototype, {
constructor : {
configurable : true,
enumerable : true,
value : Square,
writable : true
}
});
// call the supertype method
Square.prototype.toString = function() {
var text = Rectangle.prototype.toString.call(this);
return text.replace("Rectangle", "Square");
};